Theme colors can set the tone or brand consistency of a website, and being able to select and publish colors within the WordPress Customize Panel makes picking options super-convenient and easy. The method below is based on using CSS variables throughout the stylesheet to simplify color selection by using the :root
pseudo-class, and setting the key color variables and default values for each. Note that the customizer.php code can likely be condensed and refined further, and I will update this post as needed.
A simplified version of the CSS looks like this.
:root {
--bg-color: #000000;
--txt-color: #ffffff;
--hvr-color: #00ffff;
--block-color: #1a1a1a;
--std-brdr: 1px;
}
body {
color: var(--txt-color);
background: var(--bg-color);
}
a {
color: var(--txt-color);
}
a:hover {
color: var(--hvr-color);
}
code {
color: var(--hvr-color);
background-color: var(--block-color);
}
Once the stylesheet is complete using CSS variables like above, the next step is to include a new theme file in the functions.php file like this.
include( get_template_directory() . '/customizer.php' );
Finally, create a new file named customizer.php
in the theme’s directory, with the PHP code below saved to it.
<?php
function add_customizer( $wp_customize ) {
$wp_customize->add_section( 'custom_color_section', array(
'title' => 'Colors'
) );
$wp_customize->add_setting( 'body_bg_color', array(
'type' => 'option',
'default' => '#000000',
'transport' => 'refresh',
'sanitize_callback' => 'sanitize_hex_color'
) );
$wp_customize->add_control( new WP_Customize_Color_Control( $wp_customize, 'body_bg_color', array(
'label' => 'Background Color',
'section' => 'custom_color_section',
'description' => 'Change body background color.',
'settings' => 'body_bg_color'
) ) );
$wp_customize->add_setting( 'body_txt_color', array(
'type' => 'option',
'default' => '#FFFFFF',
'transport' => 'refresh',
'sanitize_callback' => 'sanitize_hex_color'
) );
$wp_customize->add_control( new WP_Customize_Color_Control( $wp_customize, 'body_txt_color', array(
'label' => 'Text Color',
'section' => 'custom_color_section',
'description' => 'Change body text color.',
'settings' => 'body_txt_color'
) ) );
$wp_customize->add_setting( 'body_acc_color', array(
'type' => 'option',
'default' => '#00FFFF',
'transport' => 'refresh',
'sanitize_callback' => 'sanitize_hex_color'
) );
$wp_customize->add_control( new WP_Customize_Color_Control( $wp_customize, 'body_acc_color', array(
'label' => 'Accent Color',
'section' => 'custom_color_section',
'description' => 'Change body accent/hover color.',
'settings' => 'body_acc_color'
) ) );
$wp_customize->add_setting( 'body_block_color', array(
'type' => 'option',
'default' => '#1A1A1A',
'transport' => 'refresh',
'sanitize_callback' => 'sanitize_hex_color'
) );
$wp_customize->add_control( new WP_Customize_Color_Control( $wp_customize, 'body_block_color', array(
'label' => 'Block Background Color',
'section' => 'custom_color_section',
'description' => 'Change code block and mobile menu background,and media outline color.',
'settings' => 'body_block_color'
) ) );
}
add_action( 'customize_register', 'add_customizer' );
function custom_color_style() {
if( get_option( 'body_bg_color' ) ) {
?>
<style type="text/css">:root{--bg-color:<?php echo get_option( 'body_bg_color' ); ?>;}</style>
<?php
}
if( get_option( 'body_txt_color' ) ) {
?>
<style type="text/css">:root{--txt-color:<?php echo get_option( 'body_txt_color' ); ?>;}</style>
<?php
}
if( get_option( 'body_acc_color' ) ) {
?>
<style type="text/css">:root{--hvr-color:<?php echo get_option( 'body_acc_color' ); ?>;}</style>
<?php
}
if( get_option( 'body_block_color' ) ) {
?>
<style type="text/css">:root{--block-color:<?php echo get_option( 'body_block_color' ); ?>;}</style>
<?php
}
}
add_action( 'wp_head', 'custom_color_style', 8 );
Note that the formatting in the custom_color_style()
section has extra spaces and tab spacing removed for a cleaner result in the head tag of the site.
Now there will be a “Colors” option in the WordPress Customize Panel, and colors can be selected and published there.
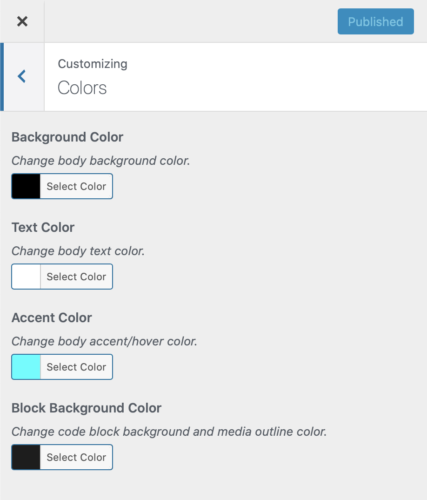